CS475: Computer Networks - Security and Privacy
Activity Goals
The goals of this activity are:- To explain the need for security and privacy protocols since these were not built into the Internet originally
- To describe the functionality of protocols used to implement authentication, authorization, non-repudiation
- To explain the role of cryptography in security and privacy, as well as its underlying algorithms
- To explain and execute the process for public-key cryptography
- To explain how public key cryptography is used to generate a digital signature that enforces non-repudiation
Supplemental Reading
Feel free to visit these resources for supplemental background reading material.The Activity
Directions
Consider the activity models and answer the questions provided. First reflect on these questions on your own briefly, before discussing and comparing your thoughts with your group. Appoint one member of your group to discuss your findings with the class, and the rest of the group should help that member prepare their response. Answer each question individually from the activity on the Class Activity Questions discussion board. After class, think about the questions in the reflective prompt and respond to those individually in your notebook. Report out on areas of disagreement or items for which you and your group identified alternative approaches. Write down and report out questions you encountered along the way for group discussion.Model 1: Public-Key Cryptography
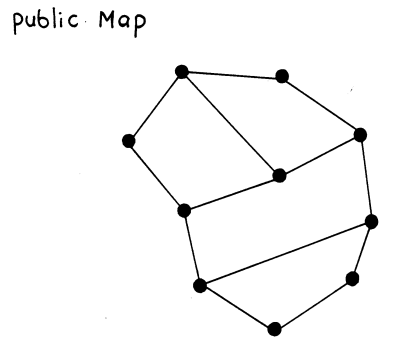
Questions
- Choose a secret numeric value that you want to send securely to a partner.
- Fill in the nodes of the public map with integers that add up to the secret value you chose.
- For each node, replace the value with the sum of itself plus the original value found in the adjacent neighbors.
- Send this graph to your partner. Can your partner figure out the value without any help (probably not!)?
- Your partner (and only your partner) should look at the Private Map (see below), which highlights the nodes that should be added together to obtain the value.
- How did this work? If every student used a unique "map," how could you use this approach to send data securely to anyone?
Model 2: Digital Signatures
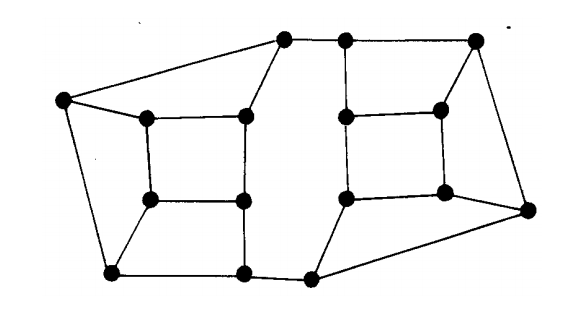
Questions
- If I encrypt something using someone's private map, who can decode it?
- If I encrypt something using my own public map, who can decode it?
- How could you use these maps to ensure that a particular person actually sent a particular value?
- How can I use this approach to ensure that the data that I sent was not altered along the way?
Model 3: SSL Certificates
const express = require('express')
const https = require('https')
const app = express();
// Usual routes
app.get('/test', (req, res) => {
res.send("Hello World!");
});
const sslOptions = {
key: fs.readFileSync('./private_key.pem'),
cert: fs.readFileSync('./certificate_chain.pem'),
ca: [
fs.readFileSync('./cert_authority.cer') //,
// ...
],
ciphers: [
"ECDHE-RSA-AES128-SHA256",
"DHE-RSA-AES128-SHA256",
"AES128-GCM-SHA256",
"RC4",
"HIGH",
"!MD5",
"!aNULL"
].join(':'),
};
const httpsServer = https.createServer(sslOptions, app);
httpsServer.listen(8443, () => {
console.log("HTTPS Running");
});
// I suggest omitting this, otherwise you have a route that can be invoked in clear text!
const httpServer = http.createServer(app);
httpServer.listen(8080, () => {
console.log("HTTP Running");
});
Questions
- What is an SSL Certificate Chain?
- What is a Certificate Authority?
- Using this command, generate and use your own SSL certificate:
openssl genrsa -out private_key.pem && openssl req -new -key private_key.pem -out csr.pem && openssl x509 -req -days 9999 -in csr.pem -signkey private_key.pem -out certificate_chain.pem
. Add these to a node.js program and invoke an endpoint over https. - Did you get a warning from your browser and, if so, why?
Embedded Code Environment
You can try out some code examples in this embedded development environment! To share this with someone else, first have one member of your group make a small change to the file, then click "Open in Repl.it". Log into your Repl.it account (or create one if needed), and click the "Share" button at the top right. Note that some embedded Repl.it projects have multiple source files; you can see those by clicking the file icon on the left navigation bar of the embedded code frame. Share the link that opens up with your group members. Remember only to do this for partner/group activities!Model 4: Signing of a Public Key by a Certificate Authority
Questions
- Although you can self-sign a certificate, why might it be more authoritative to have a trusted third party validate your identity and sign your key to form a certificate?
Model 5: SSL Handshake and Encryption
Questions
- Is the public/private key from the SSL certificate actually used to encrypt data between the client and server? Why or why not? If not, what is used instead?
Model 6: Transport Layer Security (TLS)
Questions
- How do the two peers agree upon the cipher algorithm to use?
- How do the two peers exchange their keys? How can they exchange keys securely?
- How might we prevent someone from re-ordering our encrypted packets, or from observing encrypted traffic and "replaying" it by resubmitting the traffic on our behalf?
Model 7: IPSec for Virtual Private Networks (VPN)
Questions
- What is the difference between using IPSec in Transport mode as opposed to Tunnel mode?
- Why might a tunnel be used in conjunction with an encrypted connection to provide Virtual Private Networking? Note that this is sometimes implemented with the Layer 2 Transport Protocol (L2TP) over IPSec
Model 8: Firewalls
Questions
- What are some sensible rules to block traffic, except that traffic originated by you, or incoming traffic to certain applications?
- What would you do if you wanted to allow an exception to these rules for a particular connection?
The CS Unplugged Materials are shared by CSUnplugged under a Creative Commons BY-NC-SA 4.0 License.
The Private Map for this activity can be found here and here