CS173: Intro to Computer Science - Conditionals
Activity Goals
The goals of this activity are:- To be able to write an
if
statement - To be able to write an
else
statement - To design boolean expressions for conditionals
- To combine the
if
andelse
blocks to form conditionals that utilize theelse if
construct - To implement complex conditional statements using boolean expression operators
Warmup
How might you improve thisif
statement: if(grade == 89.5) { ... }
?How would you fix this one:
if(grade > 79.5 && < 90) { ... }
The Activity
Directions
Consider the activity models and answer the questions provided. First reflect on these questions on your own briefly, before discussing and comparing your thoughts with your group. Appoint one member of your group to discuss your findings with the class, and the rest of the group should help that member prepare their response. Answer each question individually from the activity, and compare with your group to prepare for our whole-class discussion. After class, think about the questions in the reflective prompt and respond to those individually in your notebook. Report out on areas of disagreement or items for which you and your group identified alternative approaches. Write down and report out questions you encountered along the way for group discussion.Model 1: Declaring Variables within a Conditional
Questions
- What is wrong with the program above? How can you fix it?
- What would you say is the "scope" of the
total
variable? In other words, in what code block is the variable defined for use?
Model 2: Putting It All Together: Implementing a Venn Diagram
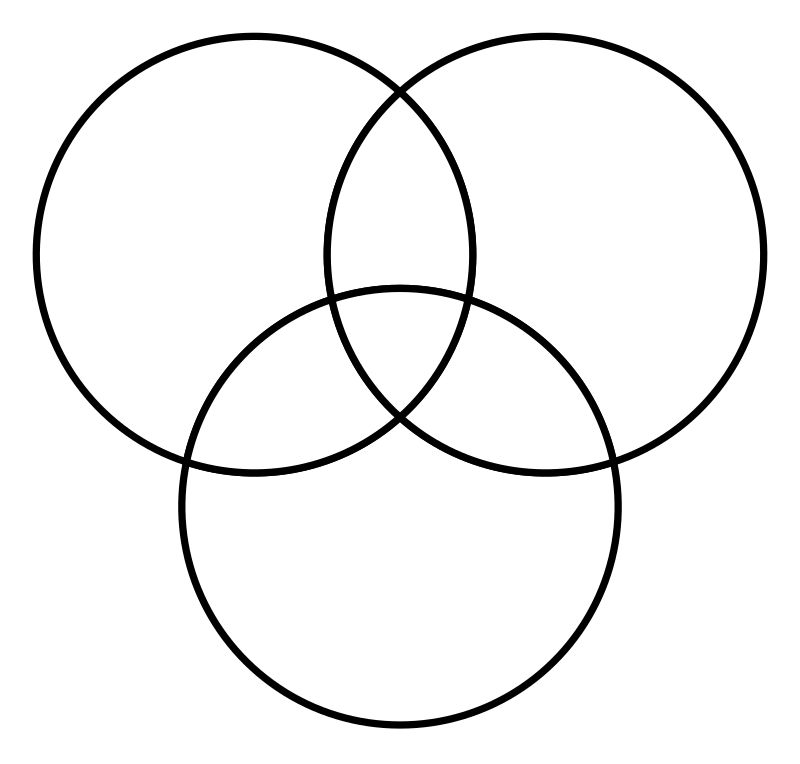
Questions
- Make up a 3-way Venn Diagram of your choosing; you can look one up on the Internet if you wish.
- Label the three large circles "A", "B", and "C". In each of the 7 regions within the Venn Diagram, which elements are true and which are false?
- Write a series of
if
statements that may useelse
andelse if
blocks that print out the different states of your Venn Diagram. There are a few ways to go about this, so we will discuss and compare approaches as a class.
Embedded Code Environment
You can try out some code examples in this embedded development environment! To share this with someone else, first have one member of your group make a small change to the file, then click "Open in Repl.it". Log into your Repl.it account (or create one if needed), and click the "Share" button at the top right. Note that some embedded Repl.it projects have multiple source files; you can see those by clicking the file icon on the left navigation bar of the embedded code frame. Share the link that opens up with your group members. Remember only to do this for partner/group activities!Model 3: Using Flow Charts to Observe Conditional Program Flow
Questions
- Draw a flowchart diagram that illustrates the control flow of your Venn Diagram program.
- Draw a flowchart of a conditional that checks if your grade is within range for each letter grade in the class.