CS173: Intro to Computer Science - Classes
Activity Goals
The goals of this activity are:- To be able to explain that classes provide methods and variables that represent complex nouns or ideas as a collection of data and functionality.
- To be able to differentiate between a class and an object of that type.
- To be able to invoke constructors using the
new
keyword.
Supplemental Reading
Feel free to visit these resources for supplemental background reading material.The Activity
Directions
Consider the activity models and answer the questions provided. First reflect on these questions on your own briefly, before discussing and comparing your thoughts with your group. Appoint one member of your group to take notes for the group, and appoint another member to discuss your findings with the class. After class, think about the questions in the reflective prompt and respond to those individually. Report out on areas of disagreement or items for which you and your group identified alternative approaches. Write down and report out questions you encountered along the way for group discussion.Model 1: Objects and Classes
Questions
- Consider the code example in the embedded frame, and the visualization of the class structures in the model. What do you notice about the name of the class and the file in which it is saved?
- What data does a
Book
contain? - How is each
Book
instantiated inmain()
? - Other editions of The Great Gatsby were released with 180 pages. What would you change in the code to reflect this?
- What would you write to print the author and title of each of these books?
Embedded Code Environment
You can try out some code examples in this embedded development environment! To share this with someone else, first have one member of your group make a small change to the file, then click "Open in Repl.it". Log into your Repl.it account (or create one if needed), and click the "Share" button at the top right. Note that some embedded Repl.it projects have multiple source files; you can see those by clicking the file icon on the left navigation bar of the embedded code frame. Share the link that opens up with your group members. Remember only to do this for partner/group activities!Model 2: Static Methods
Consider the program in the embedded frame.
Questions
- What does the
static
keyword mean?
Embedded Code Environment
You can try out some code examples in this embedded development environment! To share this with someone else, first have one member of your group make a small change to the file, then click "Open in Repl.it". Log into your Repl.it account (or create one if needed), and click the "Share" button at the top right. Note that some embedded Repl.it projects have multiple source files; you can see those by clicking the file icon on the left navigation bar of the embedded code frame. Share the link that opens up with your group members. Remember only to do this for partner/group activities!Model 3: Constructors
Consider the program in the embedded frame.
Questions
- What do you like better about this version of the program as opposed to the prior one?
- What are the values of the underlying object variables (“fields”) title, author, and pages for the Book objects
b1
,b2
,b3
, andb4
? - What happens if you set
b3 = b2
and then manipulate the fields ofb2
orb3
? - What do you think the
this
keyword means? - Why do you think we put underscore characters in the input parameters to the constructor, like
_pages
?
Embedded Code Environment
You can try out some code examples in this embedded development environment! To share this with someone else, first have one member of your group make a small change to the file, then click "Open in Repl.it". Log into your Repl.it account (or create one if needed), and click the "Share" button at the top right. Note that some embedded Repl.it projects have multiple source files; you can see those by clicking the file icon on the left navigation bar of the embedded code frame. Share the link that opens up with your group members. Remember only to do this for partner/group activities!Model 4: Creating and Accessing Arrays of Objects
Recall from our earlier examples that we had to create a separate Book object variable for each Book we wanted to create. We can simplify things by creating a single variable that refers to the whole collection. This is called an array. They allow us to leverage loops to quickly iterate over a collection of values or objects.
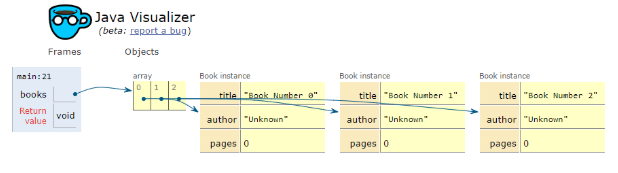
Questions
- What is the size of the
books
array? - What are the indices of the
books
array? - Why does the array use
.length
, when a String uses.length()
, to report its size? - What is the advantage of allocating an array in a loop, when the book titles may need to be set individually?